Manchmal ist es eine Herausforderung Akkus in den Helis unterzubringen, ohne dass sich Steckverbinder verfangen oder die Balancerkabel in Mitleidenschaft gezogen werden. Stickpacks können hier ein Lösung sein, welche Ihre Sichtweise auf den Heli völlig verändert. Nicht immer sind Stickpacks in der gewünschten Konfiguration erhältlich. Dieses Tutorial beschreibt, wie man sich kurzerhand selbst einen baut, der in Passform und Ausführung industriell hergestellten Stickpacks ähnelt.
Spuren zu diesem Tutorial finden sich noch in den Tiefen des Internets. Das Orginal entstammt einem RC Heli Nation Beitrag von Cris Trebbi (aka SynergyAero) vom Team SAB Goblin USA aus April 2014. Den englischen Originaltext habe ich sinngemäß übersetzt, an der ein oder anderen Stelle ergänzt oder angepasst und hoffe dass er auch für Andere hilfreich ist, die sich überlegen, einen Lipo-Stickpack selbst herzustellen.
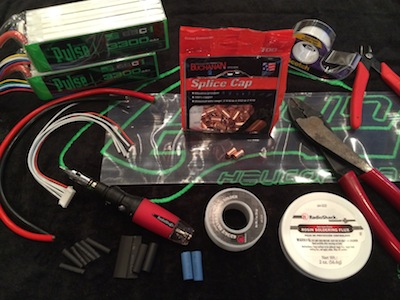
Folgende Teile werden benötigt:
- Zwei Batterien mit gleichen, technischen Spezifikationen (insbesondere hinsichtlich der nutzbaren Kapazität)
- Hochwertige, feinadrige Silikonlitze zur Verlängerung des originalen Anschlusskabels (üblicherweise 10awg)
- Verlängerungen für Balance-Stecker
- Verschiedene Größen von Schrumpfschläuchen
- Lötverbinder
- Transparentes Klebeband
- Lötzinn und Flussmittel
- Großer durchsichtiger Schrumpfschlauch zum Umwickeln des fertigen Stickpacks
Außerdem werden folgende Werkzeuge benötigt:
- Lötpistole oder Lötkolben
- Crimpzange (für nicht isolierte Stecker)
- Drahtschneider/-abisolierer
- Heißluftpistole
Zunächst sind die Hauptstromkabel, sowie die Balanceranschlusskabel der Packs zu verlängern. Anschließend können die Akkupacks hintereinander platziert werden und die gesamte Verkabelung gesichert werden. Abschließend sind beide Akkus in einem großen Schrumpfschlauch einzuschweißen.
Als Erstes müssen Sie die Hauptstromkabel des einen Akkupacks mit einem hochwertigen, feinadrigen Kabel verlängern. Dieses Kabel sollte einen vergleichbaren Querschnitt haben wie die Originalkabel der Akkus (üblicherweise 10awg).
*** Jeweils nur EIN Kabel verlängern, um zu vermeiden, dass sich freiliegende Drähte berühren, was unangenehme Folgen haben könnte!
Achten Sie darauf, dass das Minuskabel am Ende gut zu isolieren, um einen versehentlichen Kurzschluss beim Arbeiten am Pluskabel sicher zu verhindern.
Ein Stück Isolierung sowohl vom Pluskabel der Batterie als auch vom Verlängerungskabel entfernen. Nur geringfügig mehr abisolieren, als in die Lötzone des Lötverbinders passt.
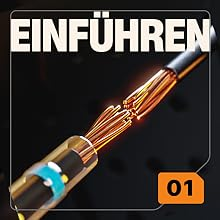
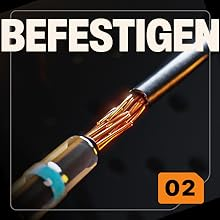
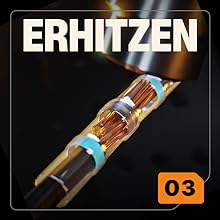
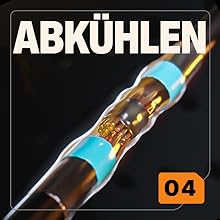
- Die Enden der Kabel abisolieren und den Lötverbinder über eines der Kabel schieben.
- Die Enden der beiden abisolierten Drähte zusammenführen und den Lötverbinder mittig über die Verbindungsstelle ausrichten.
- Mit der Heißluftpistole vorsichtig den Lötverbinder erhitzen, bis das Lötzinn im Inneren schmilzt und der Lötverbinder sich eng um die Kabel schmiegt.
- Sobald das Lötzinn geschmolzen ist, entfernen Sie die Heißluftpistole und lassen die Verbindung vollständig abkühlen. Dadurch härten Lötzinn und Kleber aus und es entsteht eine starke und zuverlässige Kabel-Verbindung.
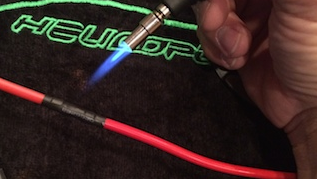
Sobald die Verbindung abgekühlt ist, als zusätzlichen Schutz einen Schrumpfschlauch hinzufügen.
Das Ende des verlängerten Pluskabels mit Isolierband oder Schrumpfschlauch isolieren. Die gleiche gleichen Schritte am Minuskabel wiederholen, um auch dieses zu verlängern.
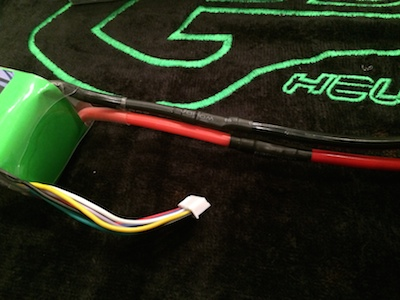
Dabei das Minuskabel soweit kürzen, dass die Kabelverbindungen später nicht nebeneinander liegen, um einen Kurzschluss auf Grund von Vibrationen oder Abnutzung zu verhindern.
Verlängern des Balancerkabels
Im nächsten Schritt ist das Balancerkabel des hinteren Akkupacks zu verlängern, hierzu verwendet man am besten ein vorkonfektioniertes Kabel.
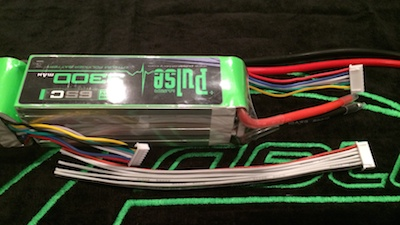
Die beiden Akkus in Reihe legen und das Balancerverlängerungskabel so ausrichten, dass der Anschluss mit dem Anschluss des vorderen Akkus übereinstimmt. Auf diese Weise kann eingeschätzt werden, wie die Verlängerung abzulängen ist, damit die Balancer-Anschlüsse des fertigen Stick-Packs später gleich lang sind. Ausgehend vom hinteren Balancerstecker etwa 1,5cm an Länge zugeben. Die Überlänge egalisiert sich durch das spätere Verdrillen und Verbinden der abisolierten Leiter.
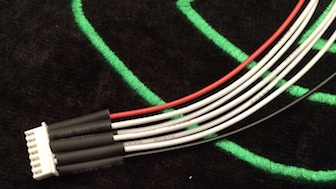
Nun einige kurze (ca. 3cm) Stücke Schrumpfschlauch mit kleinem Durchmesser abschneiden; diese werden benötigt um später die gelöteten Verbindungen zu isolieren. Am besten vor dem Löten auf die Leiter schieben, damit man sich später nicht ärgert.
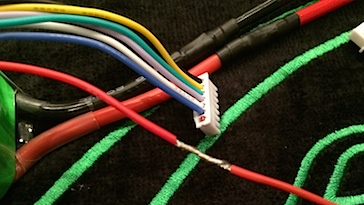
Schneiden Sie das erste Balancerkabel vom ursprünglichen Anschluss ab, und entfernen Sie etwa 1cm der Isolierung. Vom Verlängerungskabel die gleiche Länge an Isolierung entfernen.
Achten Sie darauf, dass Sie die richtigen Drähte verwenden!
Die Kabel zunächst im 90-Grad-Winkel kreuzen und dann beide Enden gleichzeitig verdrillen; dadurch erhält man eine gute Verdrillung, die sich beim anschließenden Löten nicht auflöst.
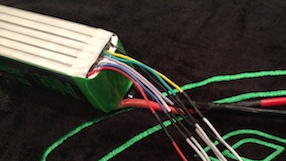
Diesen Schritt für jeweils EINEN Leiter wiederholen, bis alle Ausgleichsleitungen verlötet und mit Schrumpfschlauch überzogen sind.
Mechanisches Verbinden der Akkupacks
Die beiden Akkus mit den Enden so aneinander legen, wie sie im fertigen Stick-Pack ausgerichtet sein sollen. Haupt- und Balancerleitungen ebenfalls passend platzieren.
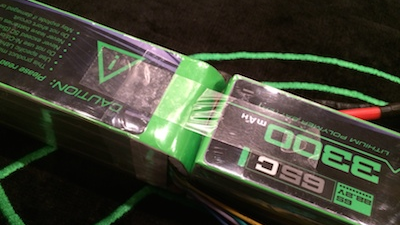
Auf dem hinteren Akku die Hälfte eine durchsichtigen Klebebands verkleben, dieses ein wenig spannen und auf den vorderen Akkupack kleben. Wurde das Klebeband gut gespannt, zieht es die beiden Akkupacks zusammen und fixiert diese.
Den Akkupack umdrehen und den Vorgang auf der Unterseite wiederholen. Das Klebeband fixiert den Pack nur vorübergehend, bis die endgültige äußere Schrumpfverpackung angebracht wird.
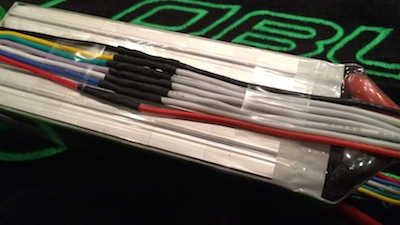
Balancer- und Akkuanschlussleitungen best möglich abflachen und mit durchsichtigem Klebeband fixieren, so dass die Leiter so eng als möglich am Akkupack anliegen. Unbeabsichtiges Verschieben beim abschließenden Schrumpfen soll dadurch vermieden werden; zudem passt ein schlanker Akku natürlich auch besser ins Modell.
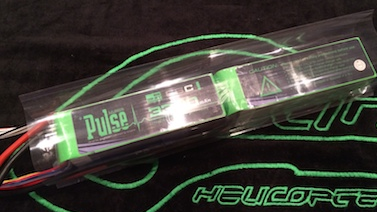
Anschließend den Akkupack umdrehen und den Vorgang für die Hauptstromkabel wiederholen. Ein großes Stück Schrumpfschlauch über die Batterien legen und so zuschneiden, dass dieser auf jeder Seite ca. 2cm über das Ende des Akkupacks hinausragt.
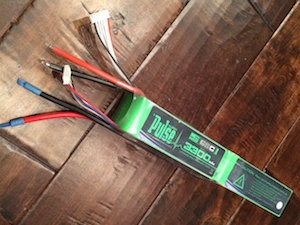
Den zugeschnitten Schrumpfschlauch über den Akkupack schieben und vorsichtig mit einer Heißluftpistole erhitzen um diesen einzuschrumpfen.
Für ein möglichst gleichmäßiges Schrumpfen mit wenig Falten, den Akkupack seitenweise vorsichtig erhitzen und dann auf die nächste Seite drehen. Dies solange wiederholen, bis der Akkupack vollständig eingeschrumpft ist.
Falls der fertige Pack zwischen den Akkus eine leichte Biegung aufweist, den Pack an dieser Stelle unter vorsichtigem Erwärmen der gekrümmten Seite durch Biegen gerade richten. Dadurch wird diese Seite gestrafft und der Akkupack bleibt dauerhaft gerade.
Wahl eines geeigneten, hochstromfähigen Stecksystems
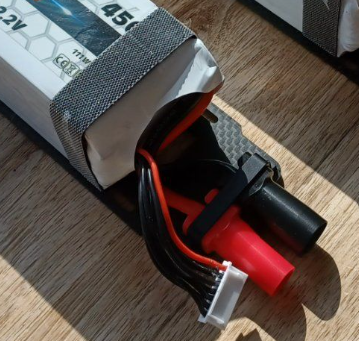
Persönlich gefällt mit das Akkuschnellwechselsystem des Nimbus 550 sehr gut.
Hierbei handelt es sich um quadratische Steckgehäuse, welche 7mm Rundkontakte des Typs AS150 aufnehmen und für 125A Dauerlast geeignet sind.